今回作成するプログラム
今回作成するプログラム
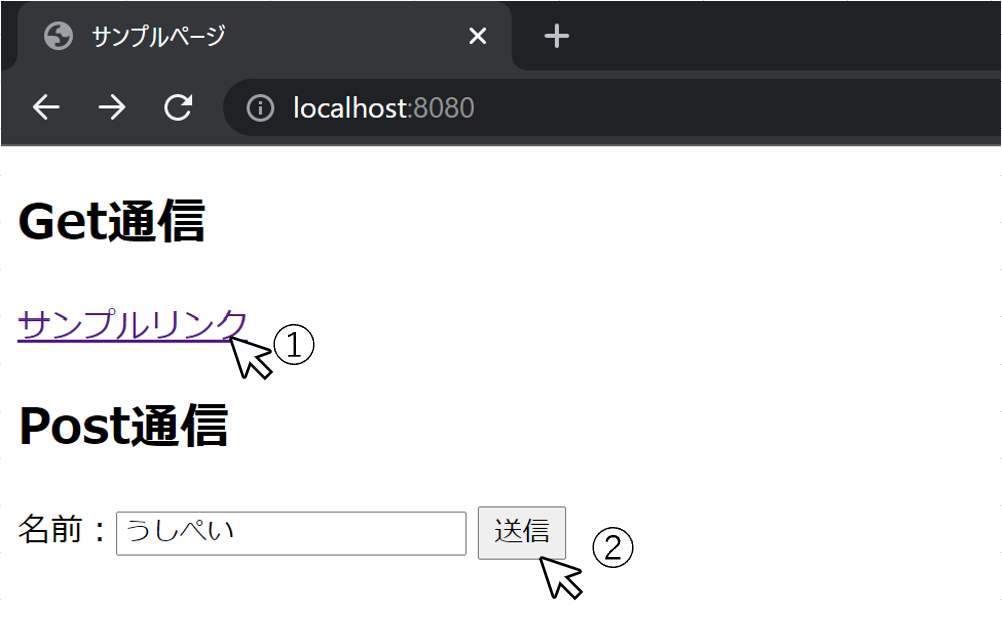
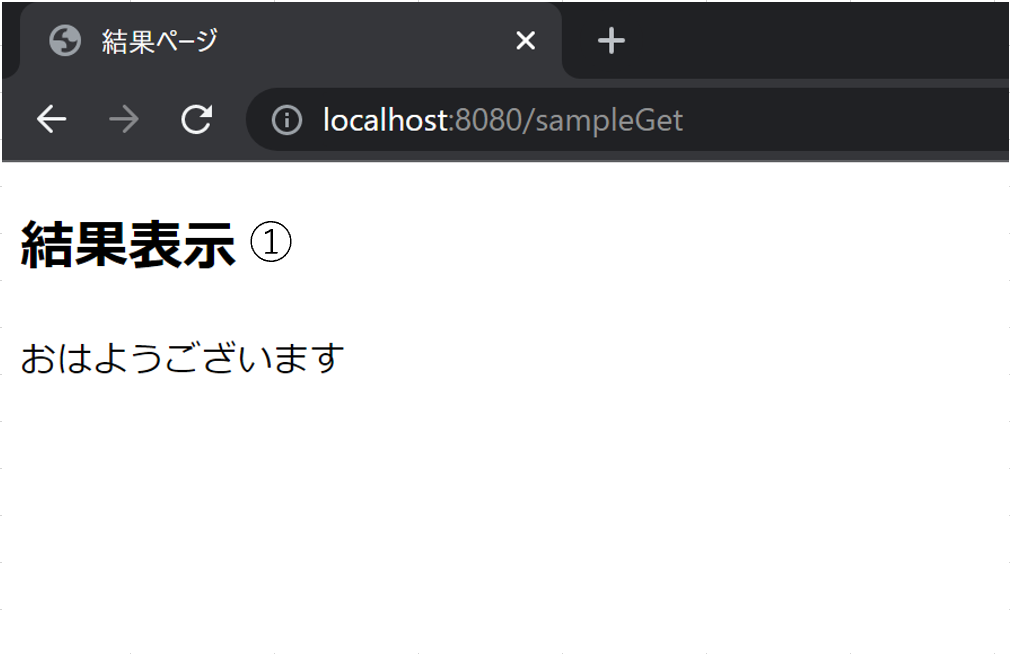
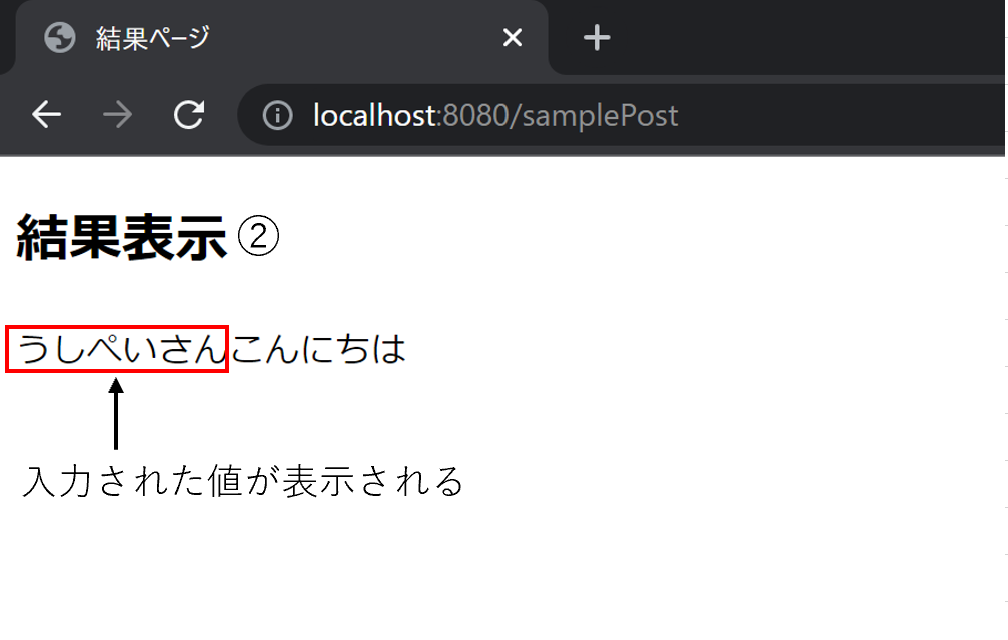
サンプルコード
<!--sample.html--> <!DOCTYPE html> <html xmlns:th="thymeleaf.org"> <head> <meta charset="UTF-8"> <title>サンプルページ</title> </head> <body> <h2>Get通信</h2> <a th:href="@{/sampleGet}">サンプルリンク</a> <h2>Post通信</h2> <form th:action="@{/samplePost}" method="Post"> 名前:<input type="text" name="name"> <input type="submit"value="送信"> </form> </body> </html>
/* * sample.java */ package com.example.demo; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestParam; @Controller public class Sample { /* * localhost:8080アクセス時にsample.htmlを返す */ @GetMapping("/") public String getSample(Model m){ return "sample"; } /* * sample.htmlで入力された値をhelloスコープに格納し * result.htmlを返す */ @PostMapping("/samplePost") public String samplePost(@RequestParam(name = "name") String name,Model m) { String hello = name + "さんこんにちは"; m.addAttribute("hello",hello); return "result"; } /* * helloスコープに文字列を格納し * result.htmlを返す */ @GetMapping("/sampleGet") public String sampleGet(Model m){ String hello= "おはようございます"; m.addAttribute("hello",hello); return "result"; } }
<!-- result.html --> <!DOCTYPE html> <html xmlns:th="thymeleaf.org"> <head> <meta charset="UTF-8"> <title>結果ページ</title> </head> <body> <h2>結果表示</h2> <p th:text="${hello}"> </body> </html>
コード解説
sample.html

tymeleafを使用するときはhtmlタグの中に上記のようにthymeleafを使用する宣言を行う
※宣言をしない場合th属性を使用することが出来ないため必ず宣言するようにする

thymeleaf内でaタグを使用する際のhref属性は以下のように記述を行う
<a th:href=”@{リンク先のパス}”>
thymeleafを使用する場合、JSPなどと異なり普通のhref属性は使用することが出来ない。
また、ページ遷移をする際などは必ず間にControllerを経由しなくてはいけないため注意する
リンクと同様に画像の参照先を指定する際は以下のように記述を行う
<img th:src=”@{画像のパス}”>

th:action=”@{フォームのリンク先}”
Sample.java

@Controllerアノテーションを宣言することでクラスをControllerとしてBeanに登録することが出来る
Beanについては別のところで解説する


th:actionの中のリンク先名を””の中に指定する
Get通信の場合はGetMappingをPost通信の場合はPostMappingを使用する
また、@RequestMappingはGet通信とPost通信の双方で使用することができる
※基本はGet通信のときはGetMappingをPost通信のときはPostMappingを使用するようにする

thymeleafから送信されたパラメータの値を受け取る際に使用する
name=”パラメータ名”を指定する
@RequestParam String パラメータ名 とすることにより(name = “パラメータ名”)を省略することができる

ModelクラスのaddAttributeメソッドを使用することでスコープに値を格納することができる
m.addAttribute(“パラメータ名”,送信する値)のように使用する送信する値にはオブジェクトを指定する
ことができる基本はDTOなどを格納して値を受け渡す

戻り値としては遷移先のHTML名を記載する
result.html

コントローラで格納した値を取り出している
th:text=”${パラメータ名}”のように使用する
インライン処理を記述する際は[[${パラメータ名}]]を使用する
以上